- Joined
- Jul 11, 2020
- Messages
- 0
- Reaction score
- 26
- Points
- 0
So, basically, I have an issue with the color codes with my custom font renderer. I don't know what's wrong.
This is my font renderer class.It is basically just the Darkstorm font renderer.
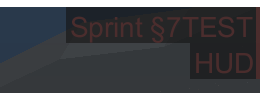
This is my font renderer class.It is basically just the Darkstorm font renderer.
Code:
/**
* This is the actual custom font.
*/
private UnicodeFont unicodeFont;
/**
* This constructor will take in a font as it parameters.
*/
public PeepFont(Font font) {
super(Wrapper.getMinecraft().gameSettings, new ResourceLocation("textures/font/ascii.png"),
Wrapper.getMinecraft().getTextureManager(), false);
unicodeFont = new UnicodeFont(font);
unicodeFont.addAsciiGlyphs();
unicodeFont.getEffects().add(new ColorEffect(Color.white));
try {
unicodeFont.loadGlyphs();
} catch (SlickException ex) {
ex.printStackTrace();
}
String alphabet = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ123456789";
FONT_HEIGHT = unicodeFont.getHeight(alphabet) / 2;
}
public int drawString(String string, int x, int y, int color) {
if(string == null)
return 0;
// glClear(256);
// glMatrixMode(GL_PROJECTION);
// glLoadIdentity();
// IntBuffer buffer = BufferUtils.createIntBuffer(16);
// glGetInteger(GL_VIEWPORT, buffer);
// glOrtho(0, buffer.get(2), buffer.get(3), 0, 1000, 3000);
// glMatrixMode(GL_MODELVIEW);
// glLoadIdentity();
// glTranslatef(0, 0, -2000);
glPushMatrix();
glScaled(0.5, 0.5, 0.5);
boolean blend = glIsEnabled(GL_BLEND);
boolean lighting = glIsEnabled(GL_LIGHTING);
boolean texture = glIsEnabled(GL_TEXTURE_2D);
if(!blend)
glEnable(GL_BLEND);
if(lighting)
glDisable(GL_LIGHTING);
if(texture)
glDisable(GL_TEXTURE_2D);
x *= 2;
y *= 2;
// glBegin(GL_LINES);
// glVertex3d(x, y, 0);
// glVertex3d(x + getStringWidth(string), y + FONT_HEIGHT, 0);
// glEnd();
unicodeFont.drawString(x, y, string, new org.newdawn.slick.Color(color));
if(texture)
glEnable(GL_TEXTURE_2D);
if(lighting)
glEnable(GL_LIGHTING);
if(!blend)
glDisable(GL_BLEND);
glPopMatrix();
return x;
}
@Override
public int drawStringWithShadow(String string, float x, float y, int color) {
return drawString(string, (int) x, (int) y, color);
}
@Override
public int getCharWidth(char c) {
return getStringWidth(Character.toString(c));
}
@Override
public int getStringWidth(String string) {
return unicodeFont.getWidth(string) / 2;
}
public int getStringHeight(String string) {
return unicodeFont.getHeight(string) / 2;
}